Building an enterprise blockchain application (part 2): demystifying the Fabric chaincode with an example
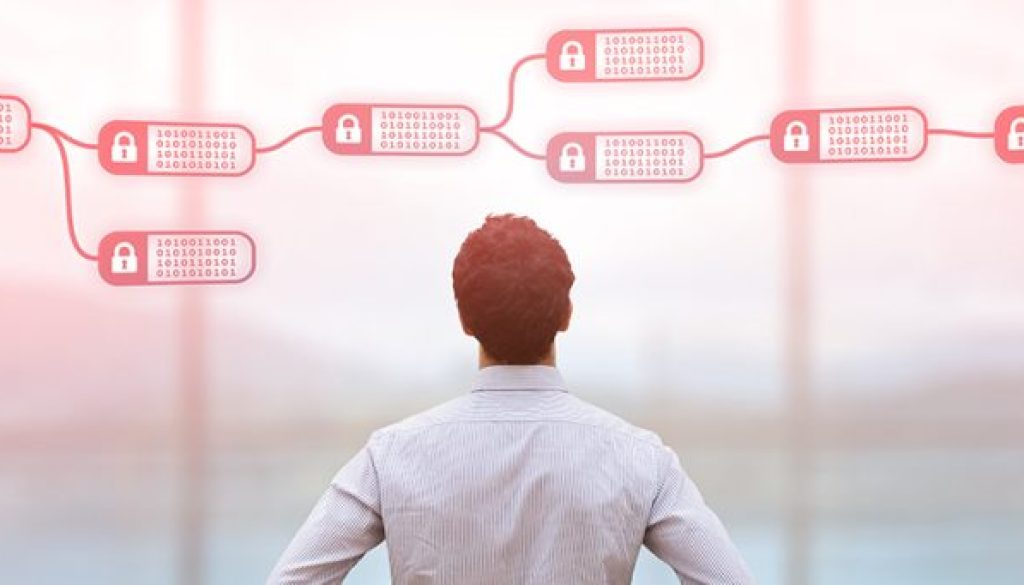
In this series of blog posts, we will take you through the steps involved in the design, architecture, and implementation of a fully functional enterprise blockchain application. We will do that by sharing content from the various development stages of a sample application that we have built for loan origination and payment on blockchain. If you follow through the end of this series, you will have a better understanding of the internal workings of the blockchain application.
For better continuity, please check out our previous post, Building an enterprise blockchain application (part 1): design and architecture.
Note: We built this application using the IBM Blockchain Platform 2.0 beta. To get started with the latest version of the IBM Blockchain Platform, you can read this blog by IBM for download instructions, which include how to get a 30-day trial.
Basics
Chaincode is the “smart contract” of Hyperledger Fabric. It encapsulates business logic and can be written in Go, Node.js, or Java. Yes, Fabric is polyglot and can provide a runtime sandbox for the chaincode to run in the previously mentioned programming languages.
A chaincode is typically used to read, write, and update the ledger of the given channel. The Fabric chaincode library provides useful APIs (shim) for chaincodes to access and update its states, get access to transaction context, and also call other chaincodes. We will walk you through these with the pseudocode of the chaincode we built for our sample application.
A chaincode that stores all loan requests
In our sample application, loanrequest.go is a simple chaincode that stores the loan applications of all applicants by applicant ID and provides functions to manage the lifecycle of those loan requests. The state stored in the ledger is actually a key-value pair with the key being the combination of applicant ID and loan request ID; the value will be the details of the specific loan request. Let’s break down the chaincode into smaller units for easier understanding:
Imports
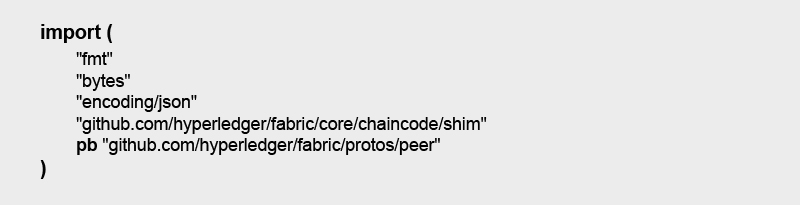
Pay attention to “github.com/hyperledger/fabric/core/chaincode/shim” because you will need this to do pretty much anything with the ledger.
Chaincode interface
All chaincodes must implement the “Chaincode” interface. This interface provides two key functions, Init and Invoke.
Init will be called once when you instantiate the chaincode or upgrade a chaincode. Use this to initialize or migrate a state.
Invoke will be called to update or query the ledger — Invoke is the entry point to your chaincode from the Fabric client SDK.
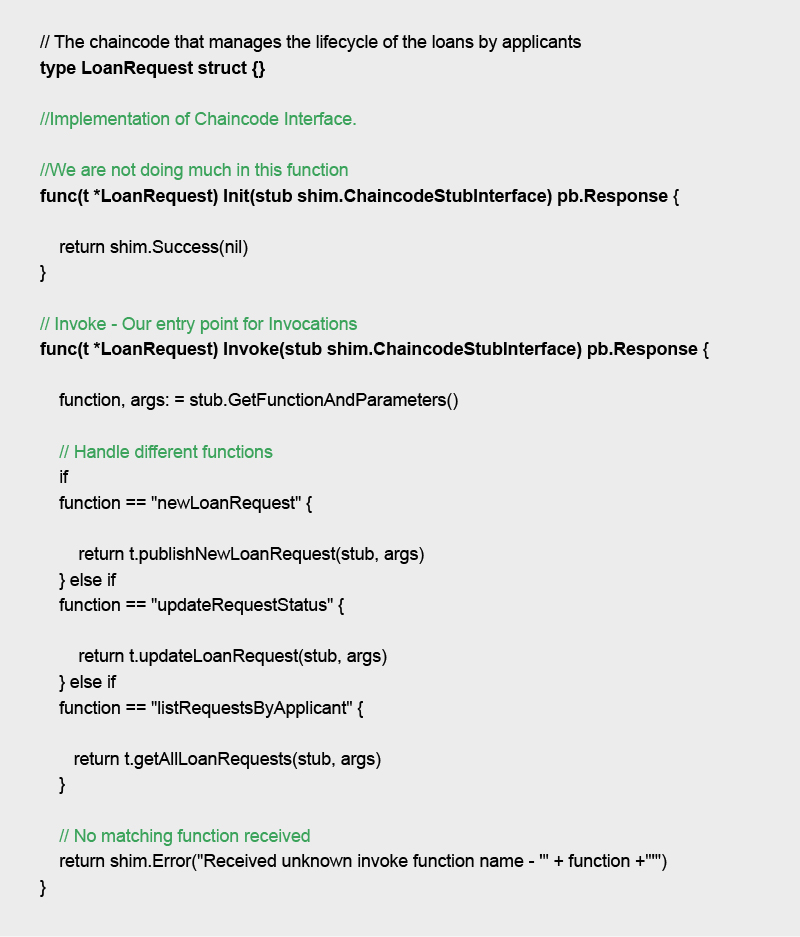
ChaincodeStubInterface.PutState
ChaincodeStubInterface provides useful functions to query and update the states in the ledger and to invoke other chaincodes. In our case, we will use PutState to store the new loan request onto the ledger in the default channel (by installing and instantiating this chaincode in the default channel) and hence making it available for all the parties in the network.
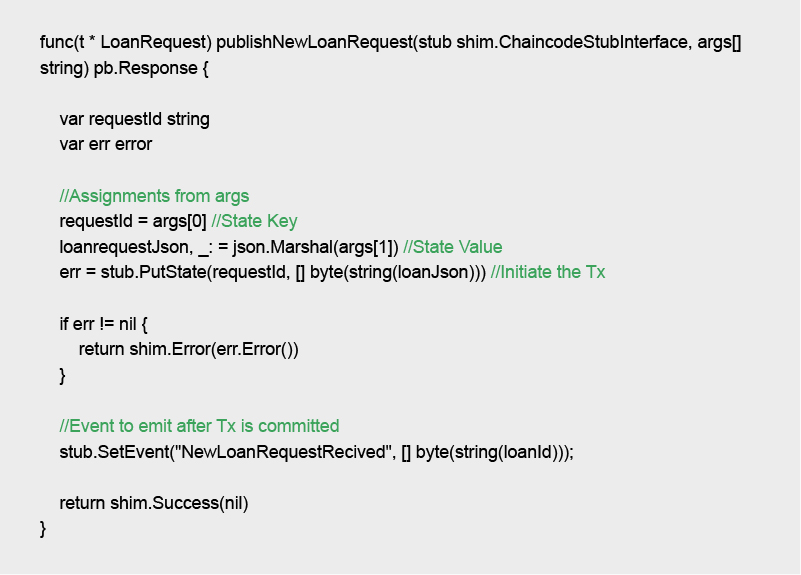
As you can see, the ledger stores a simple key-value pair with the key being request ID mapped to a JSON representing the loan request:
Pay attention to the stub.SetEvent because this is an instruction for the chaincode to emit an event when the transaction (PutState) is committed. This is the event our Event Hub will listen and react to.
Make sure you have only one event per transaction.
ChaincodeStubInterface.GetQueryResult
Use GetQueryResult to query your ledger. You can use “selector” to query the underlying CouchDB ledger like in the sample below:
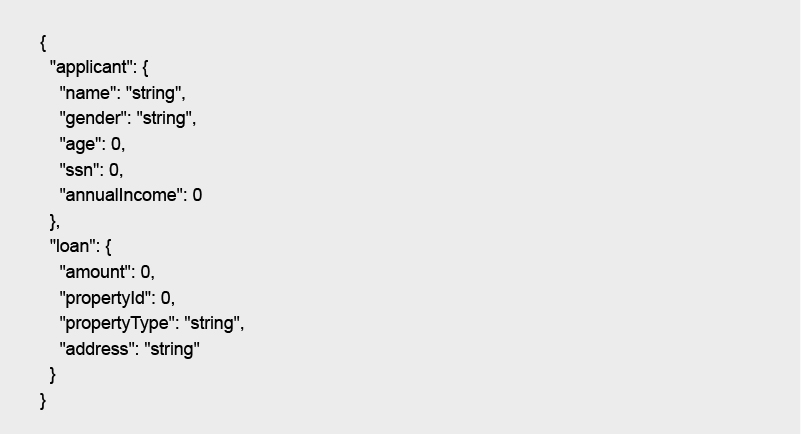
In conclusion, you can write a simple chaincode using the shim interfaces Chaincode and ChaincodeStubInterface.
We hope you found this short post useful. The next post in the series will be on the Java Fabric client SDK, showing how the chaincode functions can be invoked, integrating your enterprise applications to the blockchain behind the scenes. Hang tight — you are only a few steps away from fully understanding the internals of a fully functional blockchain application.
To see how blockchain solutions can be a business enabler for you, your customers, and your partners.